How to Find the Smallest Number in an Array in JavaScript
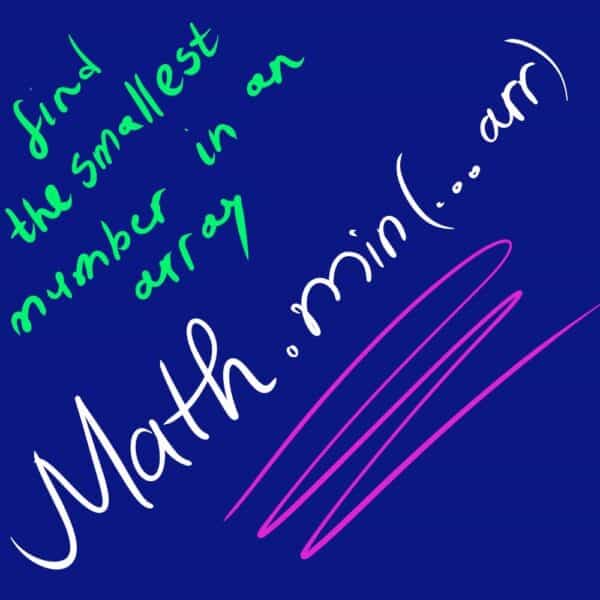
1. Find the Smallest Number in an Array in Javascript
The shortest way to find the smallest number in an array in JavaScript is to use the built-in static method Math.min(). Math.min() returns the smallest of the numbers which it takes as input parameters. The arguments are given separated with commas, for example, Math.min(1, 2, 3, 4) returns 1. We can’t pass an array as an argument, so we’ll use the spread operator (…) to split the array into separated elements and pass them to the method Math.min(…arr).
const arr = [23, -31, -40, 1, 2];
const min = Math.min(...arr);
console.log(min); // returns -40
2. Find the Smallest Number in an Array Using for Loop
2.1. Steps | Algorithm
- Create a new variable where you’ll keep the smallest number. (let min)
- Set an initial value to the newly created variable. Choose one of the following options for the initial value:
- let min = arr[0] —> the first element of the array
- let min = Infinity
- let min = Number.MAX_VALUE
- let min = Number.POSITIVE_INFINITY
- Loop through the array and compare each element with the current min value (the min variable).
- If the current item of the array is smaller than the current min value, set a new value for the min variable: min = value of the current element (the new value)
- If the current element isn’t smaller or it equals the min value – do nothing
const arr = [23, -31, -40, 1, 2];
// ---> set an initial value for min
let min = arr[0];
// or let min = Number.MAX_VALUE;
// or let min = Number.POSITIVE_INFINITY;
// or let min = Infinity;
for( let i = 0; i < arr.length; i++) {
if(arr[i] < min) { // if the current item is smaller than current min
min = arr[i]; // it will assign the value of arr[i] to the min variable
}
}
console.log(min); // returns -40
2.2. Each Iteration Explained
The below table shows what happens on each iteration of the for loop in order to find the smallest number:
iteration/i | condition | comparison | result | current min |
---|---|---|---|---|
0 | arr[0] < min | 23 < 23 | false | — |
1 | arr[1] < min | -31 < 23 | true | new min —> min = -31 |
2 | arr[2] < min | -40 < -31 | true | new min —> min = -40 |
3 | arr[3] < min | 1 < -40 | false | — |
4 | arr[4] < min | 2 < -40 | false | — |
3. Find the Smallest Number in an Array Using foreach
The steps are almost the same as in the previous example: 2.1. Steps | Algorithm. But in this case, we will use the built-in iterative foreach method. We’ll use it instead of for loop.
const arr = [23, -31, -40, 1, 2];
// ---> set an initial value for min
let min = arr[0];
// or let min = Number.MAX_VALUE;
// or let min = Number.POSITIVE_INFINITY;
// or let min = Infinity;
//loop through each element
arr.forEach(item => {
if(item < min) { // if the current item is smaller than current max
min = item; // it will assign the value of arr[i] to the min variable
}
});
console.log(min); // returns -40
In this case, the item represents the current element of the array, so instead of arr[index] in the column “condition” in the above table, we will have item (Look – 2.2. Each iteration explained ). The process is analogical.
4. Find the Smallest Number in an Array Using .sort()
The sort method takes a function as an argument. The function has two parameters, so for each step, the sort method will compare these two parameters.
4.1. Sort the Array in Ascending Order
- Sort the array in ascending order using the built-in sort() method in JavaScript.
- Get the first element of the sorted array(at index = 0).
const arr = [23, -31, -40, 1, 2];
//min
const min = arr.sort((a, b) => a - b)[0];
console.log(min); // returns -40
The below table shows how the sort method compares the arguments of the passed function (a, b). In this case, the initial value of a is the second element of the array; b is the first element of the array. If you debug the above example, you’ll get the same result. As you can see, the first number in the array is the smallest.
N | a – b | result | shift | order |
---|---|---|---|---|
0 | -31 – 23 | -54 < 0 -> negative | sort -31(a) before 23(b) | [-31, 23, -40, 1, 2] |
1 | -40 – (-31) | -9 < 0 -> negative | sort -40(a) before -31(b) | [-40, -31, 23, 1, 2] |
2 | 1 – (-40) | 41 > 0 -> positive | sort 1(a) after -40(b) | [-40, 1, -31, 23, 2] |
3 | 1 – (-31) | 32 > 0 -> positive | sort 1(a) after -31(b) | [-40, -31, 1, 23, 2] |
4 | 1 – 23 | -22 < 0 -> negative | sort 1(a) before 23(b) | [-40, -31, 1, 23, 2] |
5 | 2 – 1 | 1 > 0 -> positive | sort 2(a) after 1(b) | [-40, -31, 1, 2, 23] |
6 | 2 – 23 | -21 < 0 -> negative | sort 2(a) before 23(b) | [-40, -31, 1, 2, 23] |
4.2. Sort the Array in Descending Order
- Sort the array in descending order using the built-in sort() method in JavaScript.
- Get the last element using the length property of the array.
const arr = [23, -31, -40, 1, 2];
const min = arr.sort((a, b) => b - a)[arr.length -1];
console.log(min); // returns -40
5. Find the Smallest Number in an Array Using .reduce()
You can find the smallest number using the reduce() method and Math.min(). The reduce() method takes a callback function as a parameter and executes it on each item of the array. The result of it would be passed on to the preceding element. Reduce() takes a second parameter – an initial value. In our case, it will be Infinity.
const arr = [23, -31, -40, 1, 2];
const min = arr.reduce((a, b) => Math.min(a, b), Infinity);
console.log(min);
Let’s debug the reduce method. Each iteration is shown in the below table.
N | Math.min(a, b) | result – min |
---|---|---|
0 | Infinity, 23 | 23 |
1 | 23, -31 | -31 |
2 | -31, -40 | -40 |
3 | -40, 1 | -40 |
4 | -40, 2 | -40 |
6. Find the Smallest Number in an Array Using for of
const arr = [23, -31, -40, 1, 2];
let min = arr[0];
for (const element of arr) {
if(element < min) {
min = element;
}
}
console.log(min); // -40
Examples 2, 3, and 6 are the same but we’ve used different loops.
Conclusion
As you can see the first example is the simplest way to find the smallest number in an array in JavaScript. Furthermore, we use the ES6 spread operator which reduces our code.