Capitalize First Letter in JavaScript Make the First Letter of a String Uppercase
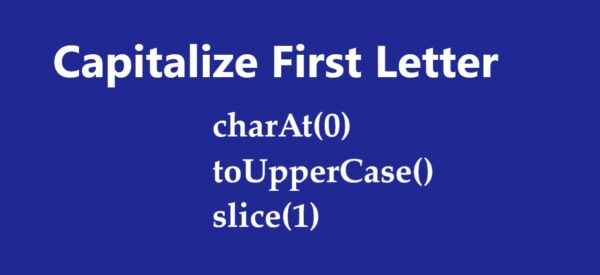
Related articles:
Capitalize the First Letter in JavaScript Using charAt(), toUpperCase() and slice()
To capitalize the first letter of a string use the charAt() method to get the first character, save it in a new variable, then call toUpperCase() to capitalize it. Extract the other part of the string in another variable using the slice() method, then concatenate the new variables.
function capitalizeFirstLetter(str) {
//gets the first letter of the string and then capitalizes it
const firstLetterToUpperCase = str.charAt(0).toUpperCase();
//gets the remaining part of the string
const otherPart = str.slice(1);
return firstLetterToUpperCase + otherPart;
}
console.log(capitalizeFirstLetter('you are incredible!')); // You are incredible!
The charAt() method returns a character at a specific index, it takes the index as an argument. toUpperCase() capitalizes the extracted letter. Then the slice(1) method extracts the remaining part of the string. It can take one or two parameters. The first indicates the substring’s starting index and the second indicates the end (the character at the specified end index is not included in the substring). If the second argument is missing, the end of the substring will be the last index of the original string.
This can be done without additional variables. Look at the short version of the previous example:
function capitalizeFirstLetter(str) {
return str.charAt(0).toUpperCase() + str.slice(1);
}
console.log(capitalizeFirstLetter('you are incredible!')); // You are incredible!
Capitalize the First Letter in JavaScript Using toUpperCase() and slice() twice
The solution is analogical: To obtain the first letter of the string, we can use the slice(0, 1) method with two arguments instead of charAt(). Index 0 is the start position of the substring, and index 1 is the end position (not included in the substring).
function capitalizeFirstLetter(str) {
return str.slice(0, 1).toUpperCase() + str.slice(1);
}
console.log(capitalizeFirstLetter('you are incredible!'));
Capitalize the First Letter in JavaScript Using toUpperCase() and substring() twice
Instead of using slice(), we can use the substring() method with the same arguments:
function capitalizeFirstLetter(str) {
return str.substring(0, 1).toUpperCase() + str.substring(1);
}
console.log(capitalizeFirstLetter('you are incredible!'));
Capitalize the First Letter in JavaScript Using split(), shift(), join() and toUpperCase()
We can convert the string to an array but it is an inefficient way to solve the problem. However, it’s not bad to practice.
function capitalizeFirstLetter(str) {
const strArr = str.split('');
const firstLetter = strArr.shift().toUpperCase();
return firstLetter + strArr.join('');
}
console.log(capitalizeFirstLetter('you are incredible!'));
The split() method separates each character of the string and makes it an element of an array. strArr.shift().toUpperCase() removes and returns the first character(element) of the array and makes it uppercase. Then gets the extracted first letter and concatenates it with the rest of the string. The join() method converts the array into a string again.