Check if a Number is a Whole Number in JavaScript
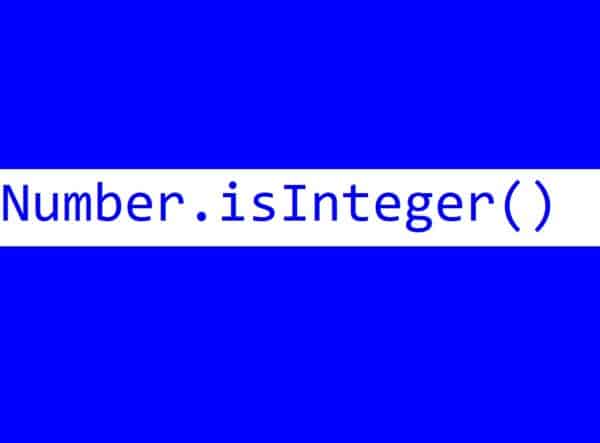
Check if a number is a whole number in JavaScript
- Use the Number.isInteger() to check if a number is a whole number.
- Check if the result of it is true or false. If it is true – the number is a whole number
function checkNumberIsWhole() {
const number = 24;
const isWholeNum = Number.isInteger(number); // pass the number as a parameter to the method
return isWholeNum;
}
console.log(checkNumberIsWhole()); // --> true
// it returns true if the number is a whole
// otherwise it will return false
The Number.isInteger() method determines whether the passed value is an integer. It returns true if the value is a whole number, and false otherwise. In the example above, we defined a variable `number` with the number you want to check and passed it to the isInteger(number) method.
Check if a number is a whole number in JavaScript using the modulus operator %
- Use the modulus operator % to check if the number is evenly divisible by 1 (remainder === 0).
- If the result is true, then the number is a whole number. Otherwise, the number is a decimal.
function checkNumberIsWhole() {
const number = 235;
return number % 1 === 0;
}
console.log(checkNumberIsWhole()); // --> true => the number is a whole number
The modulus operator (%) returns the remainder of a division operation. In the example, 235 divided by (%) 1 has a remainder of 0, number % 1 === 0
is true, so the number is an integer.
Check if a number is a whole number in JavaScript using the parseInt() function
- Pass the number you want to check as a parameter to the parseInt() function.
- Compare the returned value of it with the original number.
- If they are the same, the number is a whole number.
function checkNumberIsWhole() {
const number = 4.2;
return parseInt(number) === number;
}
console.log(checkNumberIsWhole()); //false
The parseInt() function takes a string argument and parses it to an integer value. If the argument is a decimal number, for example, a string like ‘4.2’, then the part after the decimal point will be truncated. We can compare the parsed value with the original number, and if they are equal, we can assume whether the inspected number is an integer. As you can see from the example, we can pass directly to the parseInt() function a numeric value instead of a string.
Check if a number is a whole number in JavaScript using the Math.ceil() method
- Use the Math.ceil() method to round the number up to the nearest whole number.
- Compare the rounded number with the original one.
- The number is an integer if compared values are the same.
function checkNumberIsWhole() {
const number = 20000;
return Math.ceil(number) === number;
}
console.log(checkNumberIsWhole()); //true
Check if a number is a whole number in JavaScript using the Math.round() method
- Use the Math.round() method to round the number to the nearest whole number.
- Compare the rounded number with the original one.
- The number is an integer if compared values are the same.
function checkNumberIsWhole() {
const number = 20000.23;
return Math.round(number) === number;
}
console.log(checkNumberIsWhole()); //false
Check if a number is a whole number in JavaScript using the Math.floor() method
- Use the Math.floor() method to round the number down and return a whole number.
- Compare the rounded number with the original one.
- The number is an integer if compared values are the same.
function checkNumberIsWhole() {
const number = 2345;
return Math.floor(number) === number;
}
console.log(checkNumberIsWhole()); //--> true
The difference between the last three examples is the type of rounding each method implements. Math.floor() rounds the number down, Math.ceil – up, and Math.round() returns the nearest integer.