JavaScript Data Types
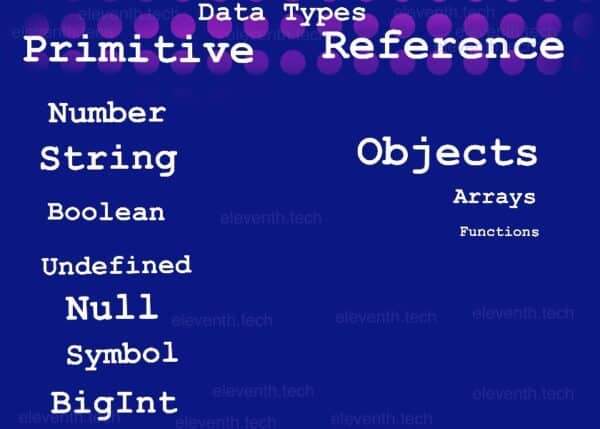
Have you ever wondered what the differences are between other programming languages and JavaScript? Sure enough, there are a lot of contrasts, but one of the main ones is how JavaScript treats its data. Let’s dive into JavaScript data types and how to use them adequately.
Javascript has 8 data types: Number, String, Null, Undefined, Boolean, BigInt, Symbol, and Object. These types can be separated into two groups which are: Primitive and Non-primitive values (also called reference data types)
What are Data Types in JS?
Primitive Data Types | Reference Data Types |
---|---|
Number: a number with or without a decimal separator | Objects: dynamic values with “key-value” pairs. Arrays and functions are also objects in JavaScript. |
Boolean: a logical value that can be true or false | |
String: a sequence of characters between single quotes, double quotes, or backticks | |
Null: an empty value explicitly assigned by the programmer | |
Undefined: an automatically assigned value by JavaScript when no value has been initialized by the programmer | |
Symbol: a unique and immutable value; often used as an Object property identifier, it prevents overwriting properties | |
BigInt: an integer value with a more extensive range than the supported Number range | |
Primitive Data Types. Where Are They Stored?
Primitive values are Number, String, Null, Undefined, Boolean, BigInt, and Symbol. These types are immutable; hence, their values cannot be changed. We can reassign a new value to an existing variable, but we cannot alter the old value itself.
In the example below, we create two variables – firstNumber and secondNumber. FirstNumber has a value of 33, and we assign the first variable to the second one, so secondNumber’s value is also 33. What will happen if we reassign a new value to firstNumber? I’m sure you know the correct answer: the variable secondNumber will not change(equals 33), but firstNumber will have a value of 44. In other words, JavaScript hasn’t changed the initial value. It has created an entirely new value (44) on the stack (don’t worry, I will give you further information about what stack is if you haven’t heard of it). Even if we reassign the second variable (secondNumer), the same process will occur – a new value will be created.

let firstNumber = 33;
let secondNumber = firstNumber;
console.log(firstNumber); // output: 33
console.log(secondNumber); // output: 33
firstNumber = 44;
console.log(firstNumber); // output: 44
console.log(secondNumber); // output: 33
secondNumber = 50;
console.log(firstNumber) // output: 44
console.log(secondNumber); // output: 50
You can see the gif I made that represents these steps, and I hope you’ll understand what immutable means. I already mentioned the stack, and I’ll give you a simple definition: a stack is a data structure used by our program to process data. All primitive values are stored there and have a fixed size, so JS knows how much room in memory a value will occupy.
What are Reference Data Types in JS?
Reference values are all types of objects – arrays, functions, and other collections in JS. Objects are dynamic values in memory – JavaScript doesn’t know their size; therefore, they can be changed. We can alter them and add new properties; thus, JavaScript will dynamically manage the memory size. Non-primitive data types are stored in a heap. The heap is another sort of data structure used by our program. Let’s look at the example below.

let obj = { type: 'computer', ram: 2 };
We declared an object “obj” that has two properties. We can see that the variable’s value stores on the stack only the memory address to the heap, so actual data (the object’s properties) is stored in the heap.
Comparing Objects with Strict Equality and Loose Equality Operators
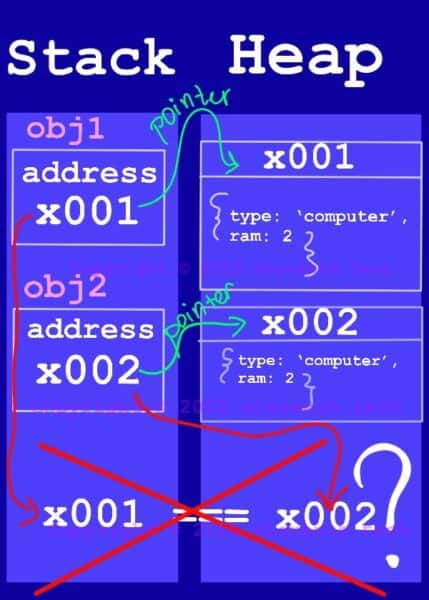
let obj1 = {
type: 'computer',
ram: 2
};
let obj2 = {
type: 'computer',
ram: 2
};
//strict equality
console.log(obj1 === obj2); //false
//loose equality
console.log(obj1 == obj2); //false
console.log(obj1.type === obj2.type); //true
console.log(obj1.ram === obj2.ram); //true
When we check objects for equality, we compare their memory addresses, not their properties. Obj1 and obj2 store two different pointers on the stack; therefore, the == operator and === operator will return false.

let firstPerson = {
name: 'Diana',
age: 22
};
let secondPerson = firstPerson;
//returns true
console.log(firstPerson === secondPerson); // true
//returns true
console.log(firstPerson == secondPerson); // true
Use strict equality and loose equality operators only if you want to determine whether two variables store the same reference(pointer) to an object.
How to check the type of a value
Use the typeof operator to determine the type of a particular value. It returns a string with the type name.
You can see in the below example that typeof null returns ‘object’, but don’t get confused – it is one of the particularities of the language. As demonstrated, when we check an array, the result is ‘object’ If you want to determine whether a value is an array, you should use the instanceof operator.
function doSomething() {}
const myName = 'Diana';
console.log(typeof myName); //string
console.log(typeof null); //object
console.log(typeof undefined); //undefined
console.log(typeof doSomething); //function
console.log(typeof 22); //number
console.log(typeof 0.0000002); //number
console.log(typeof Infinity); //number
console.log(typeof [1, 2, 3, 4, 5]); //object
console.log(typeof 'abcd'); //string
console.log(typeof new String('abcd')); //object
console.log(typeof 0); //number
console.log(typeof true); //boolean
console.log(typeof {
a: 33,
b: 33.3
}); //object
Summary
There are 8 data types. The main difference between primitive data types and objects is how JavaScript stores them in memory. The primitive values are stored on a stack, whereas objects are allocated in a heap. We can check the type of a value by using the typeof operator.