Disable a Button in Angular | 2 Easy Examples
![Disable a button in Angular - Use [disable] = "true"](https://eleventh.tech/wp-content/uploads/2022/11/DiableabuttonAngular-600x600.jpg)
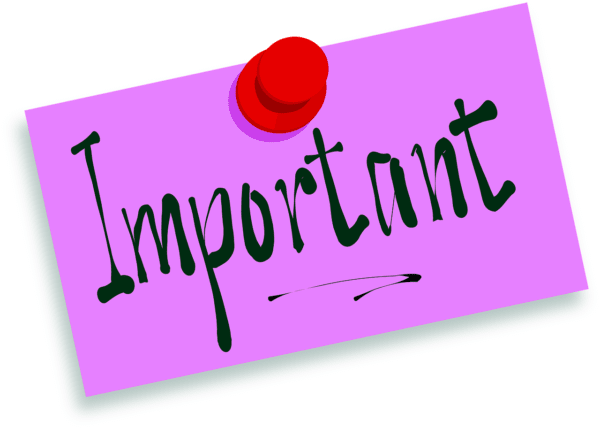
Set the disable property to true to disable a button in Angular <button [disable]=”true” …></button>
Set the disable property to false to enable a button in Angular <button [disable]=”false” …></button>
In this article, we’ll learn how to disable a button in Angular. We’ll create a simple To-Do List App with one component. The main requirements are:
- The user types a specific task in an input element and then clicks a button.
- If the input field is empty the button should be disabled and the user cannot click it.
- If the user types in the input field – the button is enabled
- When the user clicks the button, we’ll keep the data in an array
- We’ll load the array of all entered tasks in an HTML select element – each <option> element should be a task
- Another button should be presented below the list of options
- The HTML select element will have a default option ‘None’
- When the default option is selected, the second button is disabled
The below image shows how the app should work:
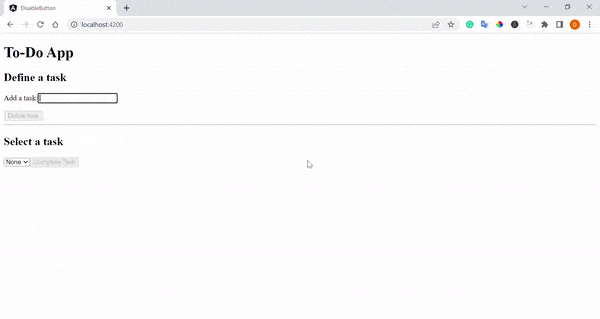
Prerequisites
Create the app
Create an app using the Angular CLI command – ng new disable-button. Delete app.component.css and app.component.spec.ts.
Create 3 properties in the class
//Code...here
//.....
export class AppComponent {
//add each entered value in the array to load it in the HTML select element
tasks: string[] = [];
//keep the entered value of the input here
inputValue = '';
//keep the current value of the HTML select element
selected = '';
//......
//Code...here
We’re creating three properties: tasks: string[], inputValue, and selected. We need the inputValue property to keep a defined task by the user. Later we’ll add it to the tasks array so we can load each task in the <select> element on the browser screen. The selected property represents the currently selected option of <select></select>. Therefore, we’ll update its value on change dynamically.
Add the necessary methods to the class
//Code ...
//we're saving each typed character in inputValue
onKeyUp(value: string) {
this.inputValue = value;
}
//we're adding the entered task to the array of tasks
onAddTask() {
this.tasks.push(this.inputValue);
this.inputValue = '';
}
//we're updating the selected property (when a change occurs in <select></select>)
onChangeOption(value: string) {
this.selected = value;
}
//Code....
The onKeyUp is responsible for handling the keyup event. When it occurs, we keep the typed data from<input> dynamically. When the user enters a character in the input field, the inputValue property of the class is updated and the button is enabled.
onAddTask() handles the click event of the button that is enabled if the input element is not empty. We save the current inputValue in the array.
onChangeOption(value: string) updates the property ‘selected’ of the class on change in the <select></select>. So we’re listening for a change event. We’ll disable the second button in the template if this property is an empty string.
1. Disable a button in Angular if an input field is an empty string
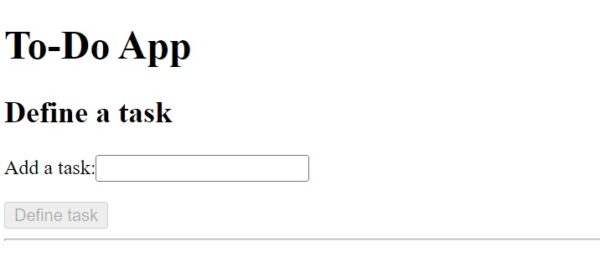
<h1> To-Do App</h1>
<h2>Define a task</h2>
<label for="chore-input">Add a task:</label>
<!-- We're defining an input field attaching keyup event to it -->
<input type="text" id="chore-input" (keyup)="onKeyUp(newTask.value)" [value]="inputValue" #newTask>
<p>{{inputValue}}</p>
<button [disabled]="!inputValue" type="button" (click)="onAddTask()">Define task</button>
<hr>
We’re listening for the keyup event and when it occurs Angular will call the onKeyUp method that updates inputValue in the class. We’re updating the value of <input></input> using property binding : [value]=”inputValue” because we want to reset it to empty string when the button is clicked. So when we change the inputValue property in the class, we actually change and the input value in the template and vice versa.
<button [disabled]=”!inputValue” type=”button” (click)=”onAddTask()”>Define task</button>
Here we’re setting [disabled]=”!inputValue” , so the expression between the double quotes evaluates to Boolean. inputValue is the class property and when it’s empty, the expression coerces to false. That’s why we need to add ! in front of it in order to disable the button.
If the button is clicked, Angular calls onAddTask – the method that keeps each entered value in an array.
2. Disable a button in Angular if the selected option of an HTML select element is a certain value
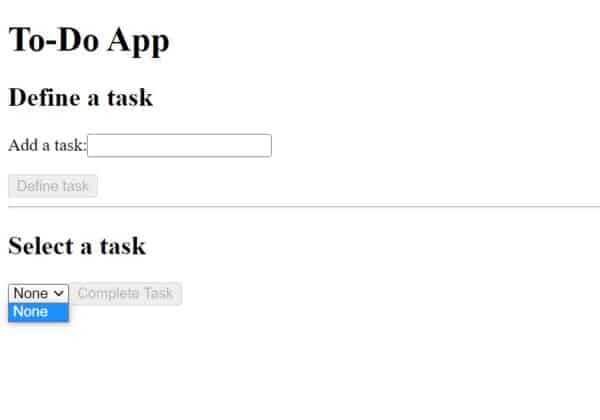
<h2>Select a task</h2>
<select #selectElement (change)="onChangeOption(selectElement.value)">
<option value="">None</option>
<option *ngFor="let task of tasks">{{ task }}</option>
</select>
<button [disabled]="!selected" type="button" >Complete Task</button>
On change the ‘selected’ property will be updated with the current value of the select. We’re passing it through the onChangeOption(selectElement.value). The first <option> element has an attribute value = “” , that way if the user selects ‘None’, the class property(selected) will be reset to an empty string.
<button [disabled]=”!selected” type=”button”>Complete Task</button>
Here we’re setting [disabled]=”!selected“ , so the expression between the double quotes evaluates to Boolean. selected is the class property and when it’s empty, the expression coerces to false. That’s why we need to add ! in front of it in order to disable the button.
Source code – disable a button in Angular
<h1> To-Do App</h1>
<h2>Define a task</h2>
<label for="chore-input">Add a task:</label>
<input type="text" id="chore-input" (keyup)="onKeyUp(newTask.value)" [value]="inputValue" #newTask>
<p>{{inputValue}}</p>
<button [disabled]="!inputValue" type="button" (click)="onAddTask()">Define task</button>
<hr>
<h2>Select a task</h2>
<select #selectElement (change)="onChangeOption(selectElement.value)">
<option value="">None</option>
<option *ngFor="let task of tasks">{{ task }}</option>
</select>
<button [disabled]="!selected" type="button" >Complete Task</button>
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css'],
})
export class AppComponent {
tasks: string[] = [];
inputValue = '';
selected = '';
onKeyUp(value: string) {
this.inputValue = value;
}
onChangeOption(value: string) {
this.selected = value;
}
onAddTask() {
this.tasks.push(this.inputValue);
this.inputValue = '';
}
}
Summary
Use the ‘disable’ property ([disabled]=”true”) to disable a button in Angular. You need to set it to true. You can add in between the quotes an expression that evaluates to boolean value.