What are Components in Angular?

To be able to understand how an Angular application works, you must first familiarize yourself with the Angular components. They are small parts through which any application is built. Let’s see what they are and what they are made of.
A component is a class associated with an HTML template, a CSS selector, and CSS styles for the template representing a part of the screen. It is the main building block for Angular applications. Components compose the application interacting with each other.
How to Create a Component
You have two options to create a component in Angular – using the Angular CLI command ‘ng generate component component-name’ or just doing it manually.
Using Angular CLI
On the console, you must be in your app directory; run the command ‘ng generate component <your-component-name>.’ It creates a new folder where all files associated with the component are stored:
- your-component-name.component.ts – the TS class
- your-component-name.component.spec.ts – testing specifications
- your-component-name.component.css – styles for the HTML template
- your-component-name.component.html – the HTML template
The Component’s Class
// --> my-component.component.ts
import { Component, OnInit } from '@angular/core';
@Component({
selector: 'app-my-component',
templateUrl: './my-component.component.html',
styleUrls: ['./my-component.component.css']
})
export class MyComponentComponent implements OnInit {
constructor() { }
ngOnInit(): void {
}
}
Angular automatically adds the required imports for your component’s class in ‘your-component-name.component.ts.’ The @Component decorator indicates that the current TypeScript class is a component and specifies the metadata necessary for Angular to find the files related to your component. The metadata is an object with the following properties:
- selector: ‘app-my-component’
- ‘templateUrl: ‘./my-component.component.html’ or template: ‘<h1>Hello World!</h1>’
- styleUrls: [‘./my-component.component.css’] or styles: [‘h1 { font-weight: normal; }’]
When Angular detects the tags <app-my-component></app-my-component>(selector) in an HTML template, it will know which component to look for. The templateUrl property defines a path to the component’s HTML template file, so Angular knows where to find it and what to render between the selector tags. If you want the HTML template to be within the component class, you can use the template property instead of templateUrl. In order to control the appearance of the component, you can add CSS styles using the styleUrls property or just styles if you don’t want separate files.
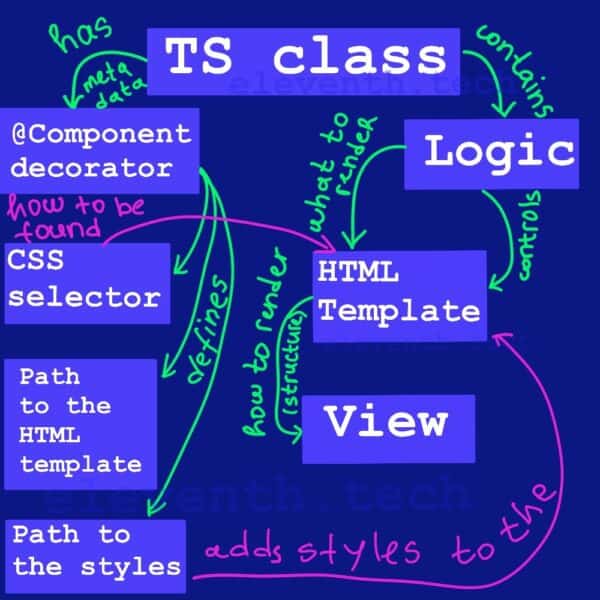
The TS class contains the logic related to the component and controls the behavior of the view through the template. It is responsible for what kind of data will be displayed, how the events will be handled, etc.
The HTML Template of the Component
The template displays the information in an HTML-structured manner and shows Angular how to render the component. In the HTML, you can also add Angular-specific syntax like structural directives(NgIf, NgForOf, NgSwitch) when you have to manipulate the DOM (to add or remove elements).
Summary
Components consist of a TypeScript class, template, and styles for the template. The class has a @Component decorator that adds metadata to it, so Angular knows which selector is related to the current template and what to render on the page.